It's quite a common requirement to add a barcode in an invoice document. In the new HTML template, you have two options to do this.
- The built-in merge field function Wp_Barcode
- Custom JavaScript
Wp_Barcode
We provide a function, Wp_Barcode, to generate barcode images, you can use it in your template as a merge field.
Usage
Here's an example to generate a QR code.
{{#Wp_Barcode}}
QR_CODE,imageWidth=100
Hello QR Code.
{{/Wp_Barcode}}
It will generate an img HTML tag like:
<img width="100" src="data:png;base64,...." />
Note:
Wp_Barcode accepts at least two lines of content.
The first line specifies the barcode options, and the other lines are barcode content.
Options
The option line format is: <code_type>[,optionName=optionValue]*.
The supported barcode types are:
- QR_CODE
- CODE_128
- CODE_39
- CODE_39_EXT
- AUSTRALIA_POST_CUSTOMER
- AUSTRALIA_POST_REPLY
- AUSTRALIA_POST_ROUTING
- AUSTRALIA_POST_REDIRECT
- ITF_14
- USPS_ONE_CODE
The following are the options
Name
|
Default
|
Description
|
imageWidth
|
300
|
Integer in pixels, the width of the generated barcode image
|
imageHeight
|
300
|
Integer in pixels, the height of the generated barcode image.
|
codeTextPosition
|
BOTTOM
|
The position of the text along with the barcode image. Options are TOP, BOTTOM, NONE.
|
Barcode With Merge Fields
You can use merge field as the content of barcode, for example:
{{#Invoice}}
{{#Wp_Barcode}}
CODE_128
*3517{{InvoiceNumber}}80*
{{/Wp_Barcode}}
{{/Invoice}}
You can also use expressions in the content as well.
{{#Invoice}}
{{#Wp_Barcode}}
CODE_128, imageWidth=200
3617{{Account.AccountNumber}}{{#Wp_Eval}}{{Amount}} * 100|Round(0){{/Wp_Eval}}0
{{/Wp_Barcode}}
{{/Invoice}}
Caveats
JavaScript
Wp_Barcode supports a list of popular barcode types, if your business requires a barcode that is not on the list, you can either submit your idea in Zuora Community, or you can leverage the JavaScript library approach.
Use HTML & JavaScript
Drag and drop to add an HTML box in your template. Copy and paste the following code:
<!-- ① -->
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/bwip-js/3.0.4/bwip-js.min.js"></script>
<!-- ② -->
<canvas id="qrcodeCanvas"></canvas>
<script>
try {
// The return value is the canvas element
let canvas = bwipjs.toCanvas('qrcodeCanvas', {
bcid: 'qrcode', // Barcode type
text: '{{Invoice.InvoiceNumber}}', // ③
});
} catch (e) {
// `e` may be a string or Error object
}
</script>
Preview the template, you will see a QR code like:
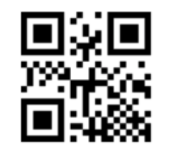
How It Works
bwip-js is a Javascript library to generate various barcode images. We just use it for demonstration purposes, you can definitely use your own JS library.
① : to load the bwip-js library when rendering the HTML.
② : Create a canvas element to draw the barcode image
③ : Keep in mind that the merge field substitution happens before the HTML rendering, so the qrcode content will be replaced into something like "INV00000028".
bwip-js supports various barcode types, see the full list and symbologies reference.
Use Your Own Fonts
The bwip-js library allows you to specify the font for the barcode text. To do that, you need to get the font file, base64 encode it,
$ base64 your_font.ttf
Note: `base64` is a CLI tool to base64 encodes the font file. You can also use online tool to do that.
And call:
bwipjs.loadFont("MICR", "<base64 encoded content of the font>");
before rendering the barcode, then you can specify the textfont option to use that font.
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/bwip-js/3.0.4/bwip-js.min.js"></script>
<canvas id="barcodeCanvas"></canvas>
<script>
bwipjs.loadFont("MICR", "<base64 encoded content of the font here>");
try {
// The return value is the canvas element
let canvas = bwipjs.toCanvas('barcodeCanvas', {
bcid: 'onecode', // Barcode type
text: '12345678901234567890',
scale: 3,
height: 10,
includetext: true,
textfont: "MICR",
textxalign: 'center',
textsize: 8
});
} catch (e) {
// `e` may be a string or Error object
}
</script>
You will see an Intelligent Mail barcode like below:
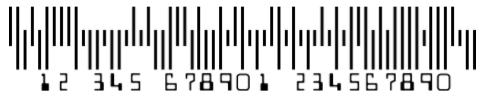
Swiss QR
Here's another sample script to generate Swiss QR code:
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/bwip-js/3.0.4/bwip-js.min.js"></script>
<canvas id="barcodeCanvas" style="width:46mm;height:46mm;"></canvas>
<script>
try {
// The return value is the canvas element
let canvas = bwipjs.toCanvas('barcodeCanvas', {
bcid: 'swissqrcode',
text: `SPC
0200
1
CH5800791123000889012
S
Robert Schneider AG
Rue du Lac
1268
2501
Biel
CH
199.95
CHF
K
Pia-Maria Rutschmann-Schnyder
Grosse Marktgasse 28
9400 Rorschach
CH
SCOR
RF18539007547034
EPD`,
scale: 2
});
} catch (e) {
// `e` may be a string or Error object
}
</script>
You can definitely replace the hard-coded text with merge fields. Preview the template, you will see an image like:
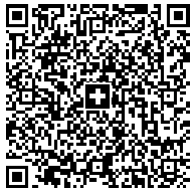
Note: Swiss QR Code specifies that the image shall be 46mm x 46mm (excluding quiet zone). Due to the difference between print and screen resolution, you will need to adjust the `scale` parameter to generate an image with the right size. Alternatively, you can use CSS style to specify the canvas size, for example:
<canvas id="barcodeCanvas" style="width:46mm;height:46mm;"></canvas>
QR Code Styling
You can also use the JavaScript approach to generate a styled QR Code. For example, add a company logo in the center of the QR Code image, or change the bar/dot styles.
In the following example, we use another 3rd-party library named
qr-code-styling.
<script type="text/javascript" src="https://unpkg.com/qr-code-styling@1.5.0/lib/qr-code-styling.js"></script>
<div id="canvas"></div>
<script type="text/javascript">
const qrCode = new QRCodeStyling({
width: 300,
height: 300,
type: "svg",
data: "https://www.zuora.com/",
image: "data:image/png;base64,<base64 encoded content>",
dotsOptions: {
color: "#0022DD",
type: "dots"
},
backgroundOptions: {
color: "#e9ebee",
},
imageOptions: {
crossOrigin: "anonymous",
margin: 20
}
});
qrCode.append(document.getElementById("canvas"));
qrCode.download({ name: "qr", extension: "svg" });
</script>
Preview, you will see a styled QR code image like:
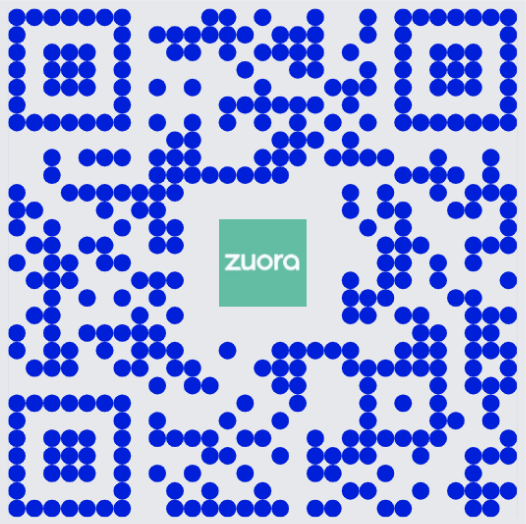
Disclaimer
The 3rd-party JavaScript library or script you use is not part of Zuora product, it's your own responsibility to implement, test, and maintain it.
Comparison
- Wp_Barcode and JavaScript approach work in different stages.
The Wp_Barcode, like other functions, is executed at the merge field binding step before HTML rendering, and the JS script works in the HTML rendering stage.
- Wp_Barcode has better performance than JS script
The HTML rendering engine needs to load the external JS library, the asset loading could be slow in case of bad network conditions.
- JS approach supports more types of barcodes than Wp_Barcode.